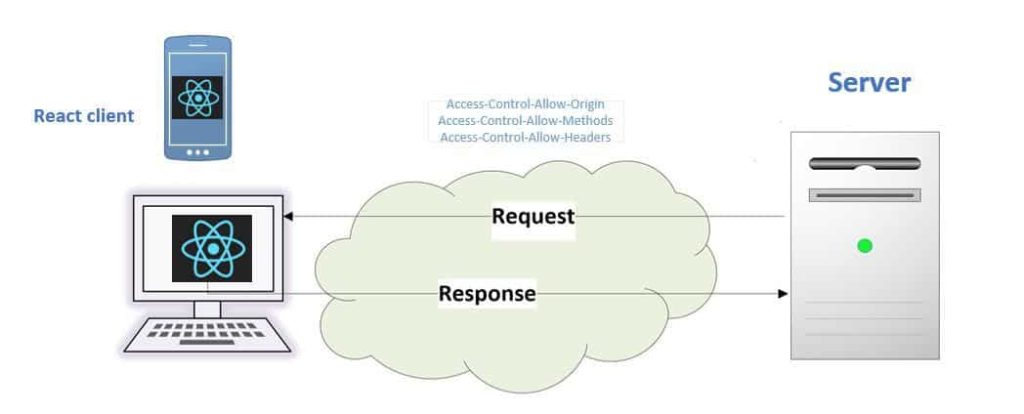
In this article, we shall see how to write React – HTTP GET, PUT, POST, and DELETE requests with easy-to-understand examples.
Below are the high-level steps that can be performed to be able to use HTTP services in React applications,
- Create a React Component ( Function-based or Class-based component -We will cover both)
- Define State objects or Hooks
- Using Fetch API
- Perform GET, PUT, POST DELETE operation
Today in this article, we will cover below aspects,
Before we get started I am assuming you already have a basic understanding of React application.
If not, kindly go through a series of articles on React,
We shall see both approaches using Function-based Component and Class-based component
Getting Started
As we saw in our last article Getting started with React, we used a function-based component where we rendered the UI with ‘Hello World’.
Here is below example of class-based Component,
Let us create your new React Application.
Fetch GET example – Using State Object
We shall be creating a CustomHttpRequest component which is a class-based component and works with Sate objects easily.
Let’s add a new CustomHttpRequest Component as below,
class CustomHttpRequest extends React.Component {
constructor(props) {
super(props)
this.state = {
date: new Date(),
name: null,
id: null,
}
}
render() {
const { name } = this.state;
const { id } = this.state;
return (
<div className="App">/
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Hello, TheCodeBuzz Community ! Current Time : {this.state.date.toLocaleDateString()}
</p>
<div className="card-body">
<p>Name: {name}</p>
<p>Id: {id} </p>
</div>
</header>
</div>
);
}
}
export default CustomHttpRequest;
In the above example, we shall be updating the State objects like name and id using HTTP GET API response.
Service API returning JSON
Let’s assume you are getting the below HTTP JSON response from Server(Node.js or ASP.NET Core or Spring )
{
"id": "d95c1c74-f1c7-480d-8ea1-621327197980",
"name": "TheCodeBuzz"
}
Add Fetch ‘GET’ method to the Component
We shall be using componentDidMount to call fetch API and we shall re-render the UI with the latest values.
componentDidMount() {
fetch('https://localhost:5001/api/values')
.then(response => response.json())
.then(data => this.setState({ name: data.name, id: data.id }))
}
componentDidMount will get called immediately after the component is mounted.
Update State object using setState
We shall be retrieving multiple values in a single call as below

Connecting API/Service endpoint
You can now ready to connect to any REST API endpoint.
I already have below API endpoint which we shall be returning as the Employee model (which we have defined as Observable above in getEmployees())
I am using the ASP.NET Core service below. However, as we know REST APIs are language agnostic, you can use Spring, Node.js, Java, or Python-based services, etc.
We are connecting to the below Service method defined @ api/values
[Route("api/[controller]")] [ApiController] public class ValuesController : ControllerBase { [HttpGet] public ActionResult<Employee> Get() { return Ok(new Employee { Id = Guid.NewGuid().ToString(), Name = "TheCodeBuzz" }); } }
CORS on Server-side
Please note your Server should allow CORS requests from your React UI domain. Please visit for more details: How to Enable CORS in ASP.NET Core REST API
Once the CORS is enabled on your server side, you shall see the GET method hit your API and give your required response.
Finally , you could see the result in the browser,
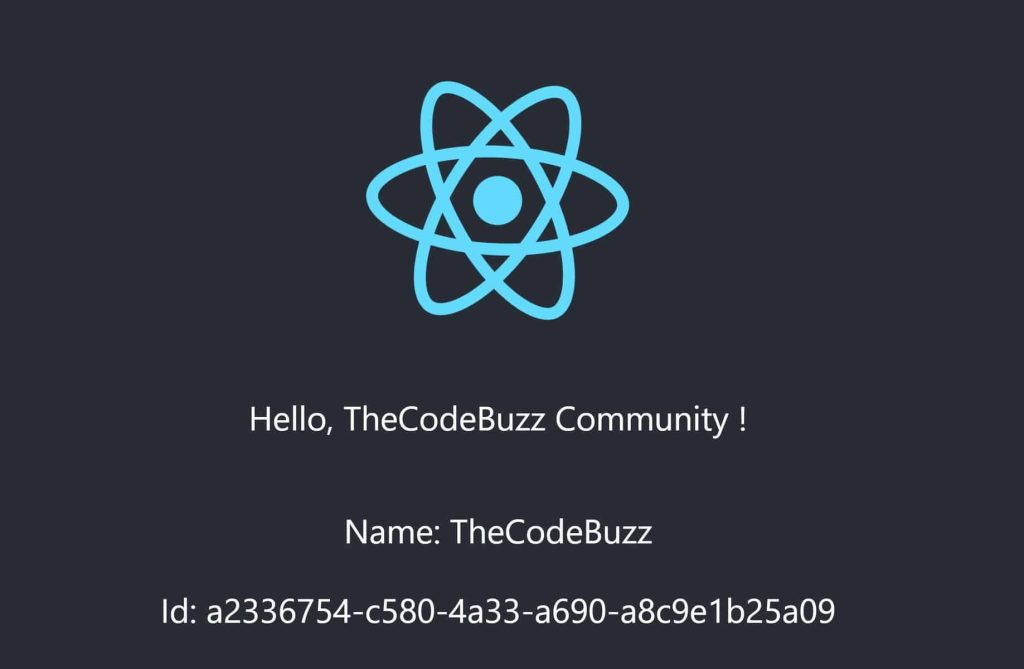
Add Http GET using Hooks and Function-based Component
Additionally, you can use Hooks and Function-based Component to call the Fetch and set the State object using Hooks defined.
Here below we are defining name and ID hooks as below,
const [name, setData] = useState(null); const [id, setId] = useState(null);
Below we are making use of useEffect() to call fetch GET API,
function HooksHttpRequest(){
const [name, setData] = useState(null);
const [id, setId] = useState(null);
useEffect(() => {
fetch('https://localhost:5001/api/values')
.then(response => response.json())
.then(data =>
{
setData(data.name);
setId(data.id)
})
},[]);
return (
<div className="App">/
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Hello, TheCodeBuzz Community !
</p>
<div className="card-body">
<p>Name: {name}</p>
<p>Id: {id} </p>
</div>
</header>
</div>
);
}
export default HooksHttpRequest;
Once run, you could see the same result in the browser.
Thats All!
For the POST method using State object and Hooks please visit the below article,
I shall be covering more on PUT and Delete with detailed examples in my next article.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we learned how to write simple React– HTTP GET, PUT, POST, and DELETE Request with easy-to-understand examples.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.